Are you tired of being blocked by Cloudflare’s security measures while trying to access websites for data scraping or automation tasks? Fear not! In this guide, we’ll explore the seamless integration of Selenium and Cloudflare in Python, empowering you to bypass Cloudflare’s protection and access your target websites effortlessly.
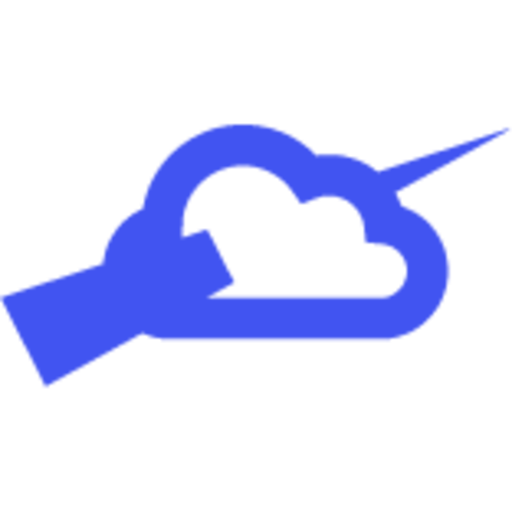
Understanding Cloudflare’s Security Measures
Cloudflare, a leading provider of internet security services, deploys various measures to protect websites from malicious traffic and bots. These measures include:
5-Second Challenge: Cloudflare presents a challenge page to users, requiring them to solve a puzzle or wait for a few seconds before accessing the website.
WAF (Web Application Firewall): Cloudflare’s WAF protects against common web-based attacks, such as SQL injection and cross-site scripting (XSS).
Turnstile CAPTCHA: Cloudflare may require users to solve a CAPTCHA before granting access to the website.
Bypassing Cloudflare with Selenium in Python
- Emulating Human Behavior
Cloudflare’s security checks are designed to distinguish between human users and bots. By using Selenium to automate browser interactions, we can emulate human behavior and bypass these checks. This includes simulating mouse movements, keystrokes, and other actions that mimic human browsing patterns. - Handling CAPTCHA Challenges
When faced with a CAPTCHA challenge, Selenium can automate the process of solving it. We can use CAPTCHA solving services or implement custom CAPTCHA solving algorithms to bypass Cloudflare’s CAPTCHA checks seamlessly. - Rotating User Agents and IP Addresses
Cloudflare may block requests based on user agents or IP addresses. Selenium allows us to dynamically rotate user agents and IP addresses, making it difficult for Cloudflare to detect and block our requests. This can be achieved by using proxy servers or IP rotation services. - Managing Cookies and Sessions
To maintain session persistence and avoid being flagged as a bot, Selenium can manage cookies and sessions effectively. By storing and reusing cookies between requests, we can ensure that our interactions with the target website appear natural and human-like.
Practical Implementation in Python
Now, let’s dive into a practical example of integrating Selenium and Cloudflare bypass techniques in Python:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
Configure Selenium WebDriver with desired options
options = webdriver.ChromeOptions()
options.add_argument(‘–disable-blink-features=AutomationControlled’)
options.add_argument(‘–disable-dev-shm-usage’)
options.add_argument(‘–no-sandbox’)
options.add_argument(‘–disable-gpu’)
options.add_argument(‘–headless’) # Optional: Run Selenium in headless mode
driver = webdriver.Chrome(options=options)
Navigate to the target website
driver.get(“https://example.com”)
Perform actions to bypass Cloudflare
(e.g., solving CAPTCHA challenges, emulating human behavior)
Extract data or perform desired tasks on the website
(e.g., scraping data, automating form submissions)
Close the Selenium WebDriver session
driver.quit()
Conclusion
By seamlessly integrating Selenium and Cloudflare bypass techniques in Python, you can overcome Cloudflare’s security measures and access your target websites without hindrance. Whether you’re scraping data for research, automating tasks, or testing web applications, mastering these techniques will empower you to achieve your goals efficiently and effectively.
So, what are you waiting for? Dive into the world of Selenium and Cloudflare integration in Python and unlock the full potential of web automation today! Happy coding!